Cognizant GenC Elevate Sample Coding Question 1
Question 1
In this article, we will discuss about Coding Question with their solution in C++, java and Python. In this Question , we have given a positive whole number n and we have to find the smallest number which has the very same digits as n and is greater than n.
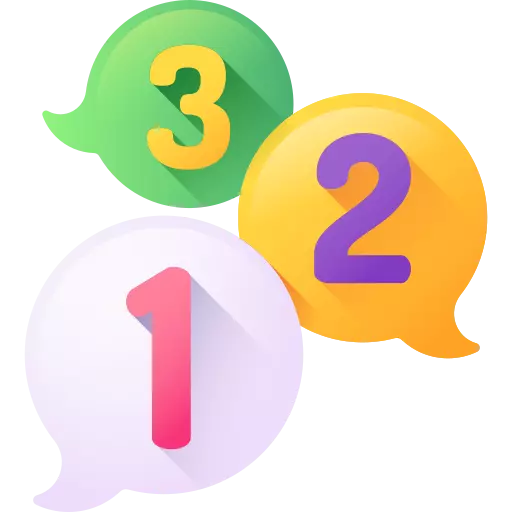
Question 1
Given a positive whole number n, find the smallest number which has the very same digits existing in the whole number n and is greater than n. In the event that no such certain number exists, return – 1.
Note that the returned number should fit in a 32-digit number, if there is a substantial answer however it doesn’t fit in a 32-bit number, return – 1.
Example 1:
Input: n = 12
Output: 21
Explanation: Using the same digit as the number of permutations, the next greatest number for 12 is 21.
Example 2:
Input: n = 21
Output: -1
Explanation: The returned integer does not fit in a 32-bit integer
#include <iostream>
#include <cstring>
#include <algorithm>
using namespace std;
void swap(char *a, char *b)
{
char temp = *a;
*a = *b;
*b = temp;
}
void findNext(char number[], int n)
{
int i, j;
for (i = n-1; i > 0; i--)
if (number[i] > number[i-1])
break;
if (i==0)
{
cout << "Next number is not possible";
return;
}
int x = number[i-1], smallest = i;
for (j = i+1; j < n; j++)
if (number[j] > x && number[j] < number[smallest])
smallest = j;
swap(&number[smallest], &number[i-1]);
sort(number + i, number + n);
cout << number;
return;
}
int main()
{
char digits[];
cin>>digits
int n = strlen(digits);
findNext(digits, n);
return 0;
}
import java.util.*;
public class Main
{
static void swap(char ar[], int i, int j)
{
char temp = ar[i];
ar[i] = ar[j];
ar[j] = temp;
}
static void findNext(char ar[], int n)
{
int i;
for (i = n - 1; i > 0; i--)
{
if (ar[i] > ar[i - 1]) {
break;
}
}
if (i == 0)
{
System.out.println("Not possible");
}
else
{
int x = ar[i - 1], min = i;
for (int j = i + 1; j < n; j++)
{
if (ar[j] > x && ar[j] < ar[min])
{
min = j;
}
}
swap(ar, i - 1, min);
Arrays.sort(ar, i, n);
for (i = 0; i < n; i++)
System.out.print(ar[i]);
}
}
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
int n =sc.nextInt();
String s = sc.next();
char digits[] = s.toCharArray();
findNext(digits, n);
}
}
arr=[]
def permute(s,ans):
n=len(s)
if (n==0):
arr.append(ans)
return
for i in range(n):
ch = s[i]
L= s[0:i]
R= s[i + 1:]
REM= L+ R
permute(REM,ans+ ch)
ans= ""
s = input()
n=len(s)
permute(s,ans)
arr=list(set(arr))
arr.sort()
ind=arr.index(s)
if(ind==len(arr)-1):
print(-1)
else:
print(arr[ind+1])
import java.util.*;
public class Main
{
static void swap(char ar[], int i, int j)
{
char temp = ar[i];
ar[i] = ar[j];
ar[j] = temp;
}
static void findNext(char ar[], int n)
{
int i;
for (i = n – 1; i > 0; i–)
{
if (ar[i] > ar[i – 1]) {
break;
}
}
if (i == 0)
{
System.out.println(“Not possible”);
}
else
{
int x = ar[i – 1], min = i;
for (int j = i + 1; j x && ar[j] < ar[min])
{
min = j;
}
}
swap(ar, i – 1, min);
Arrays.sort(ar, i, n);
for (i = 0; i < n; i++)
System.out.print(ar[i]);
}
}
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
int n =sc.nextInt();
String s = sc.next();
char digits[] = s.toCharArray();
findNext(digits, n);
}
}
mport java.util.*;
public class Solution{
public static void main(String args[])
{
Scanner s = new Scanner(System.in);
int n = s.nextInt();
int rev=0;
int num = n;
while(num!=0)
{
int temp = num%10;
rev = rev*10+ temp;
num /= 10;
} if(n>rev)
{
System.out.println(“-1”);
} if(n=0; i–)
{
if(n==i)
{
System.out.println(rev);
}
}
}
}
}
#include
using namespace std;
string solve(string s){
int j=s.length(),i;
while(j>0 && s[j]=s[j]){
j++;
}
char temp=s[i];
s[i]=s[j];
s[j]=temp;
return s;
}
int main(){
string s,ans;
cin>>s;
if(s.length()>32){
cout<<"-1";
}else{
ans=solve(s);
cout<<ans;
}
return 0;
}
digit=[]
n=int(input(“Enter a number: “))
temp=n
while n>0:
rem=n%10
digit.append(rem)
n=n//10
num=0
for i in range (len(digit)):
num=num+digit[i]
if i!=(len(digit)-1):
num=num*10
if num<=temp:
print(-1)
else:
print(num)
import itertools as it
a=input()
comb=it.permutations(a)
li=[]
for i in list(comb):
li.append(int(”.join(i)))
li.sort()
index=li.index(int(a))
if index==len(li)-1:
print(-1)
else:
print(li[index+1])
#python
from itertools import permutations
num = input()
num_list = sorted([int(i) for i in str(num)])
perm = permutations(num_list)
list = []
list_of_numbers = []
for i in perm:
set_numbers = “”.join(str(a) for a in i)
list_of_numbers.append(set_numbers)
list.append(int(set_numbers))
# print(int(set_numbers))
position = list_of_numbers.index(num)
print(list_of_numbers[position + 1])
import java.util.*;
class Practice1
{
public static void main(String[]args)
{
Scanner sc=new Scanner(System.in);
int n=sc.nextInt();
String num=Integer.toString(n);
int len=num.length();
int arr[]=new int[len];
int dup=n, d=0;
int ctr=len-1;
while(dup!=0)
{
d=dup%10;
arr[ctr]=d;
ctr–;
dup/=10;
}
ctr=len-1;
int flag=0;
while(ctr!=0)
{
if(arr[ctr]>arr[ctr-1])
{
arr[ctr]=arr[ctr]+arr[ctr-1];
arr[ctr-1]=arr[ctr]-arr[ctr-1];
arr[ctr]=arr[ctr]-arr[ctr-1];
flag++;
break;
}
ctr–;
}
for(int i=0;i<len;i++)
dup=dup*10+arr[i];
if(flag!=0)
{
System.out.println(dup);
}
else if(flag==0){
System.out.println("-1");
sc.close();
}
}
}
import java.util.*;
public class GencQ1 {
static void next(char nc[],int n)
{
int i=0;
for(i=n-1;i>0;i–)
{
if(nc[i]>nc[i-1])
break;
}
if(i==0) //if i reaches to 0 that means the array is in perfect descending order and that is the greatest element.
System.out.println(-1);
else
{
char temp;
//sorting elements from i to n inside the array
Arrays.sort(nc,i,n);//Arrays.sort(array,starting point,till the point);
//swapping the nc[i] element with the nc[i-1] element
temp =nc[i];
nc[i]=nc[i-1];
nc[i-1]=temp;
for(int l=0;l<nc.length;l++)
{
System.out.print(nc[l]);
}
}
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the elements");
String num= sc.next();
char nc[]=num.toCharArray();
int n=nc.length;
next(nc,n);
}
}
Easy Approach:
import java.util.*;
public class Solution{
public static void main(String args[])
{
Scanner s = new Scanner(System.in);
int n = s.nextInt();
int rev=0;
int num = n;
while(num!=0)
{
int temp = num%10;
rev = rev*10+ temp;
num /= 10;
}
if(n>rev)
{
System.out.println(“-1”);
}
if(n=0; i–)
{
if(n==i)
{
System.out.println(rev);
}
}
}
}
}