TCS Coding Question 2 | Given a maximum of four digit to the base 17 (10 – A …
Sweet Seventeen Problem Statement
Given a maximum of four digit to the base 17 (10 – A, 11 – B, 12 – C, 13 – D … 16 – G} as input, output its decimal value.
Test Cases
Case 1
- Input – 1A
- Expected Output – 27
Case 2
- Input – 23GF
- Expected Output – 10980
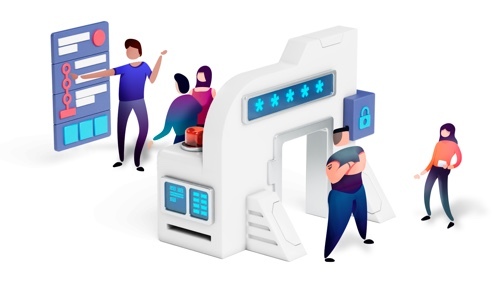
C Program
C++
Python
Java
C Program
#include <stdio.h> #include <math.h> #include <string.h> int main(){ char hex[17]; long long decimal, place; int i = 0, val, len; decimal = 0; place = 1; scanf("%s",hex); len = strlen(hex); len--; for(i = 0;hex[i]!='\0';i++) { if(hex[i]>='0'&& hex[i]<='9'){ //48 to 57 are ascii values of 0 - 9 //say value is 8 its ascii will be 56 //val = hex[i] - 48 => 56 - 48 => val = 8 val = hex[i] - 48; } else if(hex[i]>='a'&& hex[i]<='g'){ //97 to 103 are ascii values of a - g //say value is g its ascii will be 103 //val = hex[i] - 97 + 10 => 103 - 97 + 10=> val = 16 //10 is added as g value is 16 not 6 or a value is 10 not 0 val = hex[i] - 97 + 10; } else if(hex[i]>='A'&& hex[i]<='G'){ //similarly, 65 to 71 are values of A - G val = hex[i] - 65 + 10; } decimal = decimal + val * pow(17,len); len--; } printf("%lld",decimal); return 0; }
C++
#include <iostream> #include <math.h> #include <string.h> using namespace std; int main(){ char hex[17]; long long decimal, place; int i = 0, val, len; decimal = 0; place = 1; cin>> hex; len = strlen(hex); len--; for(i = 0;hex[i]!='\0';i++) { if(hex[i]>='0'&& hex[i]<='9'){ //48 to 57 are ascii values of 0 - 9 //say value is 8 its ascii will be 56 //val = hex[i] - 48 => 56 - 48 => val = 8 val = hex[i] - 48; } else if(hex[i]>='a'&& hex[i]<='g'){ //97 to 103 are ascii values of a - g //say value is g its ascii will be 103 //val = hex[i] - 97 + 10 => 103 - 97 + 10=> val = 16 //10 is added as g value is 16 not 6 or a value is 10 not 0 val = hex[i] - 97 + 10; } else if(hex[i]>='A'&& hex[i]<='G'){ //similarly, 65 to 71 are values of A - G val = hex[i] - 65 + 10; } decimal = decimal + val * pow(17,len); len--; } cout<< decimal; return 0; }
Python
'''The int() function converts the specified value into an integer number. We are using the same int() method to convert the given input. int() accepts two arguments, number and base. Base is optional and the default value is 10. In the following program we are converting to base 17''' num = str(input()) print(int(num,17))
Java
import java.util.*; public class Main { public static void main(String[] args) { HashMap<Character,Integer> hmap = new HashMap<Character,Integer>(); hmap.put('A',10); hmap.put('B',11); hmap.put('C',12); hmap.put('D',13); hmap.put('E',14); hmap.put('F',15); hmap.put('G',16); hmap.put('a',10); hmap.put('b',11); hmap.put('c',12); hmap.put('d',13); hmap.put('e',14); hmap.put('f',15); hmap.put('g',16); Scanner sin = new Scanner(System.in); String s = sin.nextLine(); long num=0; int k=0; for(int i=s.length()-1;i>=0;i--) { if((s.charAt(i)>='A'&&s.charAt(i)<='Z')||(s.charAt(i)>='a' &&s.charAt(i)<='z')) { num = num + hmap.get(s.charAt(i))*(int)Math.pow(17,k++); } else { num = num+((s.charAt(i)-'0')*(int)Math.pow(17,k++)); } } System.out.println(num); } }
Login/Signup to comment
#include
#include
int main(){
char s[20];
scanf(“%s”,s);
int len,i,j,sum,c;
for(len=0;s[len]!=’\0′;len++);
for(i=len-1,j=0;i>=0;i–,j++){
c = (int)(s[i]);
// printf(“%d “,c);
if(c>48 && c <58){
c = c -48;
sum += c*pow(17,j);
}
else{
if(s[i] == 'A') {
c= 10;
}
if(s[i] == 'B') {
c= 11;
}
if(s[i] == 'C') {
c= 12;
}
if(s[i] == 'D') {
c= 13;
}
if(s[i] == 'E') {
c= 14;
}
if(s[i] == 'F') {
c= 15;
}
if(s[i] == 'G') {
c= 16;
}
if(s[i] == 'H') {
c= 17;
}
sum += c*pow(17,j);
}
// printf("sum: %d c: %d\n", sum,c);
}
printf("%d",sum);
return 0;
}
import java.util.*;
import java.lang.Math;
public class Main
{
public static void main(String[] args) {
//System.out.println((int)’a’);
Scanner sc = new Scanner(System.in);
String str = sc.nextLine();
int len = str.length();
int sum=0;
for(int i=len-1;i>=0;i–){
if((int)str.charAt(i)>=65 && (int)str.charAt(i)=97 && (int)str.charAt(i)<=103){
sum = sum + (int)Math.pow(17,len-i-1)*((int)str.charAt(i) – 87);
//System.out.println(sum);
}else{
sum = sum + (int)Math.pow(17,len-i-1)*((int)str.charAt(i) – '0');
//System.out.println(sum);
}
}
System.out.println(sum);
}
}
#include
using namespace std;
int main()
{
string s;
cin>>s;
int mul=1,res=0;
for(int i=s.size()-1 ; i>=0;i–)
{
if(s[i]>=’A’ &&s[i]=’0′ &&s[i]<='9')
res += (s[i]-'0')*mul;
mul*=17;
}
cout<<res;
return 0;
}
#include
#include
#include
int main()
{
int i=0,val,len; char hex[20];
long decimal=0,place=1;
scanf(“%s”,hex);
len=strlen(hex)-1;
for(i=0;hex[i]!=’\0′;i++)
{
if(hex[i]>=’0’&& hex[i]=’a’&& hex[i]<='z')
val=hex[i]-97+10;
else
val=hex[i]-65+10;
decimal=decimal+val*pow(17,len);
len–;
}
printf("%ld",decimal);
return 0;
}
#taking Raw Input
userInput = raw_input()
#saving in list so that we get character by character
num = list(userInput)
#creating a dictionary and mapping
thisDict = {
“A”:10,
“B”:11,
“C”:12,
“D”:13,
“E”:14,
“F”:15,
“G”:16,
“H”:17,
“I”:18,
“J”:19,
“K”:20,
#so on…
}
power = len(num)-1
final = 0
for i in num:
if i in thisDict:
demo=thisDict[i] * 17**power
final = demo + final
power = power-1
else:
demo=int(i) * 17**power
final = demo + final
power = power-1
print(final)
——————————————–
add lowercase alphabeths to urs dictionary too.
Wow its to easy but can you please explain for python
import java.io.*;
class A{
public static void main(String args[])throws Exception{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
String s=br.readLine();
System.out.println(Integer.parseInt(s,17));
}
}
This much code is enough for this question.
python solution is not clear
#include
using namespace std;
int main() {
string s; cin>>s;
int l = s.size(); int tl=l;
//cout<<l<<endl;
int num=0;
for(int i=0; i<tl; i++){
if(s[i]=='0' || s[i]=='1' || s[i]=='2' || s[i]=='3' || s[i]=='4' || s[i]=='5' || s[i]=='6' || s[i]=='7' || s[i]=='8' || s[i]=='9'){
num = num + ((int)s[i]-48)*pow(17,l-1) ;
}
else{
char ts = s[i]; int p=0;
if(ts=='A') p=10;
else if(ts=='B') p=11;
else if(ts=='C') p=12;
else if(ts=='D') p=13;
else if(ts=='E') p=14;
else if(ts=='F') p=15;
else if(ts=='G') p=16;
num=num+p*pow(17,l-1);
}
l–;
}
cout<<num;
}
class Question2 {
public static void main(String[] args) {
String number = “1G”; // Number
System.out.println(Integer.valueOf(number, 17)); //17 is base
}
}
You can use
System.out.println(Integer.parseInt(number,17),10);
Edited: you can use it too
System.out.println(Integer.toString(Integer.parseInt(number,17),10);
#include
#include
#include
int main()
{
int l,t,val,d=0,n=0,i;
char s[32];
scanf(“%s”,s);
l=strlen(s)-1;
for(i=l;i>=0;i–)
{
t=s[i];
if(t>=48&&t=97&&t=65&&t<=90)
{
val=t-55;
}
d=d+val*pow(17,n);
n++;
}
printf("%d",d);
}
for(int i=s.length()-1;i>=0;i–)
{
if((s.charAt(i)>=’A’&&s.charAt(i)=’a’ &&s.charAt(i)<='z'))
{
num = num + hmap.get(s.charAt(i))*(int)Math.pow(17,k++);
}
else
{
num = num+((s.charAt(i)-'0')*(int)Math.pow(17,k++));
}
can any one explian how is it working