TCS Coding Question 3
Oddly Even Problem Statement
Given a maximum of 100 digit numbers as input, find the difference between the sum of odd and even position digits
Test Cases
Case 1
- Input: 4567
- Expected Output: 2
Explanation : Odd positions are 4 and 6 as they are pos: 1 and pos: 3, both have sum 10. Similarly, 5 and 7 are at even positions pos: 2 and pos: 4 with sum 12. Thus, difference is 12 – 10 = 2
Case 2
- Input: 5476
- Expected Output: 2
Case 3
- Input: 9834698765123
- Expected Output: 1
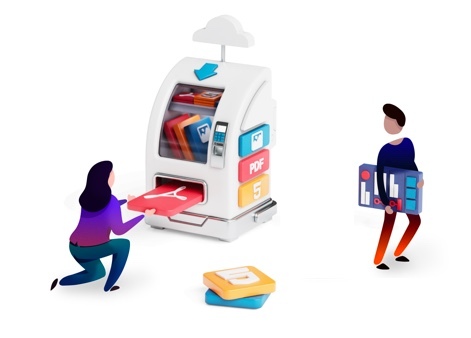
Solution
(When using Strings as input)
C
C++
Python
Java
C
#include <stdio.h> #include <string.h> #include <stdlib.h> int main() { int a = 0,b = 0,i = 0, n; char num[100]; printf("Enter the number:"); scanf("%s",num); //get the input up to 100 digit n = strlen(num); while(n>0) { if(i==0) //add even digits when no of digit is even and vise versa { a+=num[n-1]-48; n--; i=1; } else //add odd digits when no of digit is even and vice versa { b+=num[n-1]-48; n--; i=0; } } printf("%d",abs(a-b)); //print the difference of odd and even return 0; }
C++
#include <iostream> #include <string.h> #include <stdlib.h>
using namespace std; int main() { int a = 0,b = 0,i = 0, n; char num[100]; cout<< "Enter the number:"; cin>> num; //get the input up to 100 digit n = strlen(num); while(n>0) { if(i==0) //add even digits when no of digit is even and vise versa { a+=num[n-1]-48; n--; i=1; } else //add odd digits when no of digit is even and vice versa { b+=num[n-1]-48; n--; i=0; } } cout<< abs(a-b); //print the difference of odd and even return 0; }
Python
num = [int(d) for d in str(input("Enter the number:"))] even,odd = 0,0 for i in range(0,len(num)): if i % 2 ==0: even = even + num[i] else: odd = odd + num[i] print(abs(odd-even)) # code contributed by Shubhanshu Arya PrepInsta Placement Cell Student
Java
import java.util.*; public class Main { public static void main(String[] args) { Scanner sin = new Scanner(System.in); String s=sin.nextLine(); long num = 0, num1 = 0; num=num + s.charAt(0)-'0'; for(int i=1;i<s.length();i++) { if(i%2==0) num = num + s.charAt(i)-'0'; else num1 = num1 + s.charAt(i)-'0'; } System.out.println(Math.abs(num-num1)); } }
Solution
(When using long long as input)
C
C++
Python
C
#include<stdio.h> #include<string.h> #include <stdlib.h> int main() { int odd = 0,even = 0,i = 0, n,diff; long long num; scanf("%lld",&num); //get the input up to 100 digit while(num != 0){ if(i%2==0){ even = even + num%10; num = num/10; i++; } else{ odd = odd + num%10; num = num/10; i++; } } printf("%d",abs(odd - even)); return 0; }
C++
#include<iostream> #include<string.h> #include <stdlib.h>
using namespace std;
int main() { int odd = 0,even = 0,i = 0, n,diff; long long num; cin>>num; //get the input up to 100 digit while(num != 0){ if(i%2==0){ even = even + num%10; num = num/10; i++; } else{ odd = odd + num%10; num = num/10; i++; } } cout<< abs(odd - even); return 0; }
Python
num = [int(d) for d in str(input("Enter the number:"))] even,odd = 0,0 for i in range(0,len(num)): if i % 2 ==0: even = even + num[i] else: odd = odd + num[i] print(abs(odd-even))