Question 5
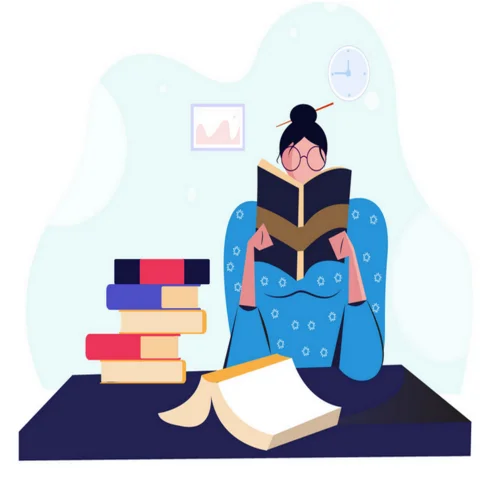
Pangram Checking
Given a string check if it is Pangram or not. A pangram is a sentence containing every letter in the English Alphabet.
Examples : The quick brown fox jumps over the lazy dog ” is a Pangram [Contains all the characters from ‘a’ to ‘z’]
“The quick brown fox jumps over the dog” is not a Pangram [Doesn’t contains all the characters from ‘a’ to ‘z’, as ‘l’, ‘z’, ‘y’ are missing]
We create a mark[] array of Boolean type. We iterate through all the characters of our string and whenever we see a character we mark it. Lowercase and Uppercase are considered the same. So ‘A’ and ‘a’ are marked in index 0 and similarly ‘Z’ and ‘z’ are marked in index 25.
After iterating through all the characters we check whether all the characters are marked or not. If not then return false as this is not a pangram else return true.
Code for checking whether a string is Pangram or not
// C Program to check the given string is a pangram or not #include<stdio.h> int main() { char str[120]; int i,alphabet[26]={0},result=0; printf("Enter the String: "); scanf("%s",str); for(i=0;str[i]!='\0';i++) { if('a'<=str[i] && str[i]<='z') { result+=!alphabet[str[i]-'a']; alphabet[str[i]-'a']=1; } else if('A'<=str[i] && str[i]<='Z') { result+=!alphabet[str[i]-'A']; alphabet[str[i]-'A']=1; } } if(result==26) { printf("The String is a Pangram"); } else { printf("The String is not a Pangram"); } return 0; }
Output
Enter the String: qwertyiopasdfghjklzxcvbnm
The String is a Pangram
// Java Program to check whether a string is Panagram or not import java.util.*; class Main { // Returns true if the string is pangram else false public static boolean checkPangram (String str) { // Create a hash table to mark the characters present in the string // By default all the elements of mark would be false. boolean[]mark = new boolean[26]; int index = 0; // Traverse all characters for (int i = 0; i < str.length (); i++) { // If uppercase character, subtract 'A'to find index. if ('A' <= str.charAt (i) && str.charAt (i) <= 'Z') index = str.charAt (i) - 'A'; // If lowercase character, subtract 'a'to find index. else if ('a' <= str.charAt (i) && str.charAt (i) <= 'z') index = str.charAt (i) - 'a'; // Mark current character mark[index] = true; } // Return false if any character is unmarked for (int i = 0; i <= 25; i++) if (mark[i] == false) return (false); // If all characters were present return (true); } // Main Code public static void main (String[]args) { Scanner sc=new Scanner(System.in); System.out.println ("Enter a string"); String str=sc.next(); if (checkPangram (str) == true) System.out.print (str + " is a pangram."); else System.out.print (str + " is not a pangram."); } }
Output
Enter a string
The quick brown fox jumps over the lazy dog
The quick brown fox jumps over the lazy dog is a pangram
s=”The quick brown fox jumps over the lazy dog”
x=s.lower()
y=set(x)
if ” ” in y:
y.remove(” “)
n=len(y)
print(n)
if n==26:
print(“The String is a Pangram”)
else:
print(“The String is not a Pangram”)
my code in c++ to check the string:
#include
#include
#include
#include
using namespace std;
#include
int main()
{
string s ;
int flag = 0;
getline(cin,s);
transform(s.begin(), s.end(), s.begin(), ::tolower);
int freq[26] = {0};
for(int i =0 ;i<s.length();i++)
{
freq[s[i] – 'a']++;
}
for(int i =0 ;i<26;i++)
{
if(freq[i]0)
cout<<"The String is a Pangram";
else
cout<<"The String is not a Pangram";
return 0;
}
Python code
def ispangarm(str):
alphabets = ‘abcdefghijklmnopqrstuvwxyz’
for char in alphabets:
if char not in str.lower():
return False
return True
string = ‘qwertyuiopasdfghjklzxcvbnm’
if ispangarm(string)==True:
print(‘given string is pangram’)
else:
print(‘given string is not pangram’)
string = input().lower()
alphabets = [i for i in ‘abcdefghijklmnopqrstuvwxyz’]
counter = 0
for i in alphabets:
if i not in string:
counter = counter + 1
if counter>0:
print(‘Not a panagram’)
else:
print(‘panagram’)
n=input().lower()
n=n.replace(‘ ‘,”)
lis=[]
for i in n:
for j in i:
lis.append(j)
p=set(lis)
q=len(p)
if q==25:
print(‘The string is a Pangram’)
else:
print(‘not a pangram’)
Do it in shorter way:- 😉
—————————————————————
public static void main(String[] args) {
String str = “The quick brown fox jumps over the Lazy dog”;
boolean result = str.toLowerCase().replaceAll(“[^a-z]”, “”).replaceAll(“(.)(?=.*\\1)”, “”).length() == 26;
if(result)
System.out.println(str+” is a Pangram.”);
else
System.out.println(str+” is not a Pangram”);
}
//Coding by Arman.
——————————————————————-
public class Main {
public static void main(String[] args) {
String s = “The quick brown fox jumps over the lazy dog”;
List list = new ArrayList();
List newList = new ArrayList();
for(int i=0; i
= ‘A’ && s.charAt(i)= ‘a’ && s.charAt(i)<= 'z' )){list.add(String.valueOf(s.charAt(i)).toLowerCase());
}
}
Collections.sort(list);
newList = list.stream().distinct().collect(Collectors.toList());
if(newList.size()==26){
System.out.println("above sentence is Pangram ");
}else{
System.out.println("above sentence is not a Pangram ");
}
}
}
python code:
string=input(“enter string”).lower()
alph=”abcdefghijklmnopqrstuvwxyz”
beta=”ABCDEFGHIJKLMNOPQRSTUVWXYZ”
result=”panagram”
for i in alph:
if i not in string:
result=”not a panagram”
print(result)
#include
#include
#include
using namespace std;
bool isPangram(string s)
{
int arr[26];
for(int i = 0; i < 26; i++)
arr[i] = 0;
for(char c : s)
{
c = toupper(c);
int asciiIndex = (int) c – 65;
arr[asciiIndex]++;
}
for(int i = 0; i < 26; i++)
{
if(arr[i] == 0)
return false;
}
return true;
}
int main()
{
string s;
getline(cin,s);
if(isPangram(s))
cout <<"Yes"<<endl;
else
cout << "NO"<<endl;
return 0;
}
PYTHON CODE—
alphabets=’abcdefghijklmnopqrstuvwxyz’
n=input()
li=[]
count=0
for val in n:
if(val in alphabets and val not in li):
count+=1
li.append(val)
if(count==26):
print(‘this is Pangram’)
else:
print(‘not pangram’)
Also gets the missing characters in the string if any ..
alphabets=”abcdefghijklmnopqrstuvwxyz”
d=({})
c=0
for i in alphabets:
d[i]=0
a=input()
for i in alphabets:
if i in a:
d[i]=1
for i in alphabets:
if d[i]==0:
c+=1
print(i,end=” “)
if c==0:
print(“No missing letters”)
import java.util.Scanner;
public class Prog61
{
static int flag=0;
static int check(char ch)
{
for(int i=0;i<26;i++)
{
if(ch==(char)(i+97) || ch==(char)(i+65))
{
flag=1;
break;
}
else
{
flag=0;
}
}
if(flag==0)
{
return 0;
}
else
{
return 1;
}
}
public static void main(String args[])
{
Scanner sc=new Scanner(System.in);
String strNew=sc.nextLine();
String str1=strNew.replaceAll("//s","");
boolean var=true;
char str[]=str1.toCharArray();
int count=0;
int arr[]=new int[40];
for(int i=0;i<26;i++)
{
arr[i]=0;
}
for(int i=0;i<str.length;i++)
{
if(str[i]=’a’ || str[i]>=’A’ && str[i]<='Z'){
arr[count++]=check(str[i]);
}
else
{
i++;
}
}
//checking for pangram
for(int i=0;i<count;i++)
{
if(arr[i]==1)
{
var=true;
}
else{
var=false;
System.out.print("Not Pangram String");
break;
}
}
if(var==true)
{
System.out.print("Pangram String");
}
}
}
Thanks for contributing the code Adit
//c++ code for the same….o(n)
#include
#include
using namespace std;
int main()
{
string str = “The quick brown fox jumps over the lzy dog”;
int arr[26];
for( int i=0;i<26;i++){
arr[i]=0; }
for(int i=0;i=65 && x=97&&x<123)){
arr[x-97]=1;
}
}
int x=0;
for( int i=0;i<26;i++){
if(arr[i]==1){
x++;
}
}
if (x==26){
cout<<"pangram";
}
else{
cout<<"not";
}
return 0;
}